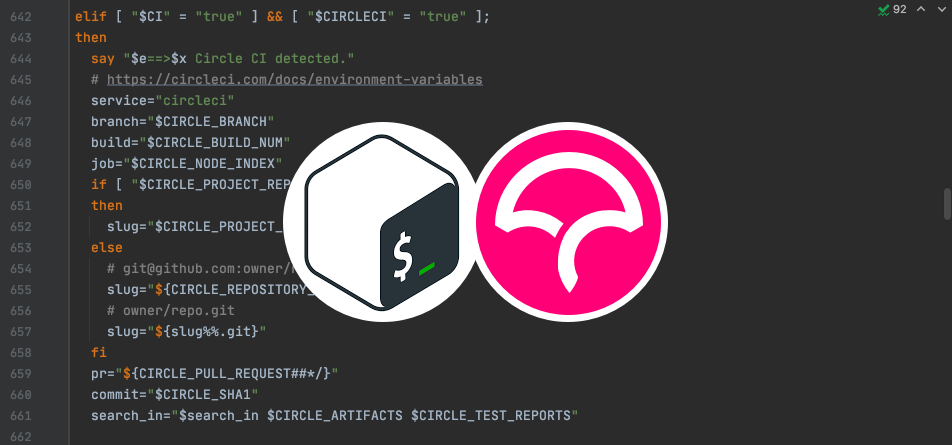
Codecov was built to work for as many languages and configurations as possible. In this post, we show you how to begin collecting coverage for your bash scripts and how to upload the reports to Codecov.
We will be using bashcov, which is based on simplecov to collect coverage metrics. We show how to do this with GitHub Actions and CircleCI, but you can use any CI/CD to upload to Codecov.
Create a base configuration for your CI/CD
In order to start using Codecov for bash scripts, you’ll need to set up your CI/CD to be compatible with Ruby and to check out your code from your git repositories. Below are boilerplate configurations we will use for GitHub Actions and CircleCI.
GitHub Actions
name: Test and coverage
on: [push, pull_request]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
with:
fetch-depth: 2
- uses: actions/setup-ruby@v1
with:
ruby-version: '2.7'
CircleCI
version: 2.1
jobs:
build:
docker:
- image: circleci/ruby:2.7
steps:
- checkout
workflow:
version: 2.1
build-test:
jobs:
- build
Add Ruby dependencies in your Gemfile
In order to start collecting coverage, you will need to add the following Ruby dependencies. You can do this by adding the below Gemfile
to the root of your project or add the bashcov
and codecov
gems to an existing spec.
Gemfile
# frozen_string_literal: true
source 'https://rubygems.org'
gem 'bashcov'
gem 'simplecov'
gem 'simplecov-cobertura'
We will also need to update our CI/CD configuration to install the dependencies after checkout.
GitHub Actions
- name: Install Ruby dependencies
run: bundle update --bundler && bundle install
CircleCI
- run:
name: Install bundler and dependencies
command: bundle update --bundler && bundle install
Include a .simplecov file
Codecov will need a coverage report that is properly formatted to be ingested. We can override the SimpleCov formatter with the simplecov-cobertura formatted.
.simplecov
require 'simplecov'
require 'simplecov-cobertura'
SimpleCov.formatter = SimpleCov::Formatter::CoberturaFormatter
Add a step to run scripts and upload coverage reports to Codecov
Now that we have a way of generating coverage reports for Codecov, we need to update our CI/CD configuration to run the script and upload them. If you are using a public repository running GitHub Actions, TravisCI, CircleCI, or Appveyor, you will need to retrieve an upload token from your Codecov repository settings page.
GitHub Actions
- name: Run script
run: bashcov <script.sh>
- name: Upload reports to Codecov
uses: codecov/codecov-action@v2
CircleCI (uses the Codecov CircleCI orb)
- run:
name: Run scripts
command: bashcov <script.sh>
- codecov/upload
Run your build!
Now we are ready to commit the following code changes and push them up to your preferred code host. Open a pull request and be sure that you have connected your CI/CD to your repository. When the build has completed, you should receive a Codecov comment and status check.
Codecov Comment
Codecov Status Checks
All of the above code snippets can be found on our example bash repository. Now that you have uploaded your first coverage report to Codecov, you can upgrade your code coverage by configuring Codecov settings, adding flags, or viewing the sunburst graph to identify code areas in need of test coverage.